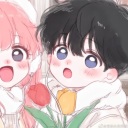
Zood by Fabra
**Changelog: Old z.lua → New z.lua** **1. Schema System** - Old: Single `BaseSchema` with manual validation. - New: Modular `Schema` objects per type (`string`, `number`, `table`, etc.), each with chainable methods. **2. Error Handling** - Old: Errors as flat string tables. - New: Errors as rich objects (`ValidationError`) with fields (`message`, `code`, `path`, `value`, etc.) and a `.format()` method for pretty-printing. **3. Custom Error Messages** - Old: Only per-schema/rule. - New: Global, per-schema, and per-rule error messages, with clear priority and formatting (including path, expected/actual values). **4. Validation API** - Old: `:parse` (throws), `:safeParse` (returns boolean, errors). - New: Same API, but `:safeParse` returns `(ok, valueOrErrorObject)`; error object is much richer. **5. Chaining & Modifiers** - Old: Limited chaining. - New: Consistent chaining for all schemas (`:min`, `:max`, `:pattern`, `:optional`, `:nullable`, `:default`, `:custom`, `:transform`). **6. Type Coercion** - Old: None. - New: `Z.coerce` helpers for string, number, boolean, with configurable truthy/falsy values. **7. Table/Array/Enum/Union** - Old: Basic, manual logic. - New: First-class support, strict/loose/strip modes, unknown key handling. **8. Performance** - New: Table pooling, pattern/message caching. **9. ComputerCraft Support** - New: Built-in schemas for peripherals, colors, sides, with validation. **10. Usage** - Use `schema:safeParse(value)` for validation. - On failure: `result.errors` (table of errors), `result:format()` (formatted string). **Summary:** The new version is more modular, extensible, and user-friendly, with robust error handling, customization, and ComputerCraft compatibility.
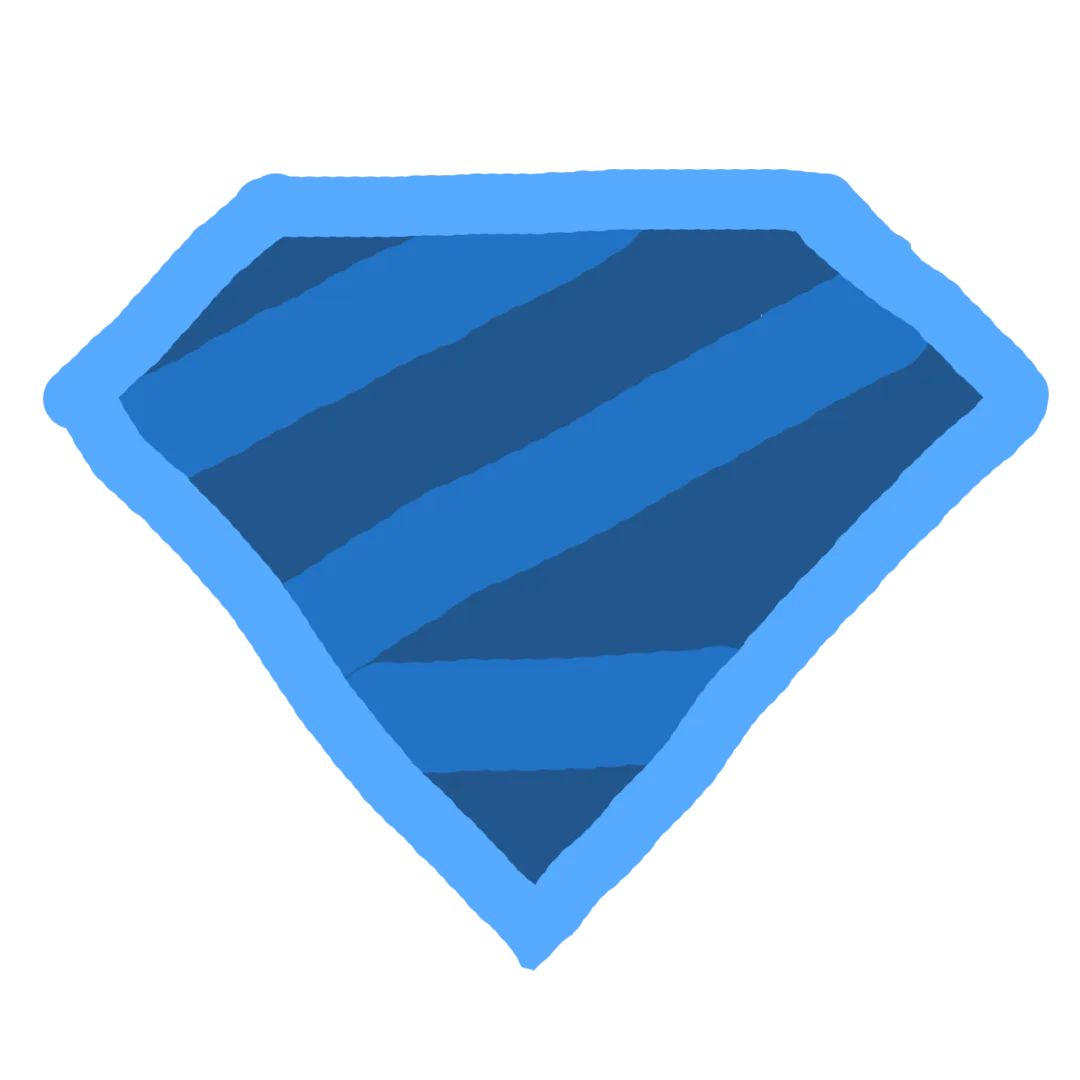
Welcome to Zood
Zood is a Lua library for ComputerCraft that provides a powerful and flexible way to validate and transform data structures. Inspired by Zod for TypeScript, Zood brings type safety and schema validation to Lua, making it easier to handle complex data in your ComputerCraft programs.
Features
- Type Validation: Ensure data matches expected types (e.g., strings, numbers, tables).
- Custom Validators: Add custom validation logic to suit your needs.
- Data Transformation: Transform data as it is validated (e.g., trim strings, convert case).
- Error Handling: Detailed error messages for debugging and validation failures.
- Schema Composition: Combine schemas to create complex validation rules.
Quick Start
Here’s a quick example of how to use Zood to validate a table:
local Z = require("z")
local schema = Z.table({
name = Z.string(),
age = Z.number():positive(),
email = Z.string():email()
})
local data = {
name = "Alice",
age = 30,
email = "[email protected]"
}
local ok, result = schema:safeParse(value)
if not ok then
-- result is a ValidationError object
print(result:format()) -- Formatted error string (all errors, nicely formatted)
for _, err in ipairs(result.errors) do
print(err.message) -- Each error message (raw)
-- err is a table with fields: message, code, path, value, expected, received, details
end
end
wget run https://pinestore.cc/d/135Git Repository
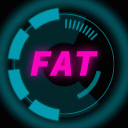
url schema does not allow direct IPs or localhost!
http://localhost
-> Valid!
http://127.0.0.1
-> Valid!
thanks for catching that.. I've updated the URL schema now